In this lesson, we explore Python While Loop. Unlike conditional statements in Python, while loops repeatedly execute a block of code as long as a specified condition remains true. This dynamic structure is perfect for scenarios where you want to iterate until a particular condition is no longer satisfied.
Python While Loop:
A “while” loop is a programming construct that repeatedly executes a block of code as long as a specified condition remains true. The loop evaluates the condition before each iteration and continues executing the code inside the loop as long as the condition is true.
Conditionals within a Loop:
Within a “while” loop, you can incorporate “if” statements to introduce conditional logic. These statements enable the program to make decisions based on certain conditions. If the condition specified in the “if” statement is true, a particular block of code is executed; otherwise, an alternative block of code can be executed.
Counting using While Loop:
A common application of a Python While loop is counting. For instance, you can initialize a counter variable and increment it within the loop, printing values as they change. This enables the loop to iterate a specific number of times.
Program: Simple Counting with While Loop
# Title: Basic Counter
count = 1
while count <= 5:
print(f"Count: {count}")
count += 1
Explanation:
- This program initializes a counter variable count to 1.
- The while loop runs as long as count is less than or equal to 5.
- Inside the loop, the current value of count is printed, and then count is incremented by 1.
Python While Loop: Break Statement:
The “break” statement is used to prematurely exit a loop, regardless of whether the loop condition is true or false. When a specified condition is met, the “break” statement terminates the loop, transferring control to the next statement after the loop.
while condition:
if some_condition:
break
Here, if “some_condition” is true, the loop stops executing, and the program proceeds to the code after the loop.
Program: Countdown with Break Statement
# Title: Countdown
counter = 10
while counter > 0:
print(f"Countdown: {counter}")
counter -= 1
if counter == 5:
print("Breaking the loop at countdown 5.")
break
Explanation:
- This program initializes a counter variable counter to 10.
- The while loop runs as long as the counter is greater than 0.
- Within the loop, printing the current value of the counter occurs, and subsequently, decrementing the counter by 1 takes place.
- There is an “if” statement to break the loop when the counter reaches 5.
These examples demonstrate the use of while loops, conditionals within loops, counting, and the break and continue statements in Python programming.
Python While Loop: Continue Statement:
Using the “continue” statement within a loop involves skipping the remaining code in the current iteration and proceeding to the next iteration of the loop. This allows for the selective execution or skipping of code based on specific conditions.
while condition:
if some_condition:
continue
In this case, if “some_condition” is true, the loop skips the subsequent code in the current iteration and proceeds to the next iteration. This offers a way to customize the flow of the loop based on certain criteria.
Program: Skip Even Numbers with Continue Statement
# Title: Skip Even Numbers
number = 1
while number <= 10:
if number % 2 == 0:
print(f"Skipping even number: {number}")
number += 1
continue
print(f"Processing odd number: {number}")
number += 1
Explanation:
- This program initializes a variable number to 1.
- The while loop runs as long as the number is less than or equal to 10.
- Inside the loop, an “if” statement checks if the current number is even (number % 2 == 0).
- If the number is even, it prints a message and continues to the next iteration using the continue statement.
- If the number is odd, it prints a message indicating processing.
Python While Loop: Problem examples
Here are 10 problem examples involving while loops, conditionals within a loop, counting using a while loop, and the use of break and continue statements:
1: Use a while loop to count from 1 to 10, printing each number.
2: Modify the previous program to skip printing even numbers.
3: Create a program that counts down from 10 to 1 using a while loop.
4: Calculate and print the sum of the first 5 natural numbers using a while loop.
5: Implement a password guessing game using a while loop. Give three tries, stop the loop when the right password is entered.
6: Write a program to calculate the factorial of a given number using a while loop.
7: Use nested while loops to print the following pattern:
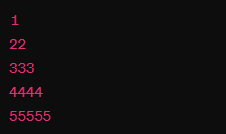
8: Creating a number guessing game using a “while” loop involves providing hints like “too high” or “too low” until the player guesses the correct number. The loop breaks once the player guesses the correct number.
9: Write a program that generates an “infinite” loop and utilizes the break statement to exit the loop after meeting a specific condition.
10: Modify the previous program to use the continue statement to skip printing even numbers in the infinite loop.