Introduction:
Today, let’s dive into the basics of conditional statements in Python using straightforward examples. We’ll explore nested conditionals, multi-way decisions, the difference between else-if and if-if statements, and the simplicity of conditional expressions. Ready? Let’s begin this learning journey!
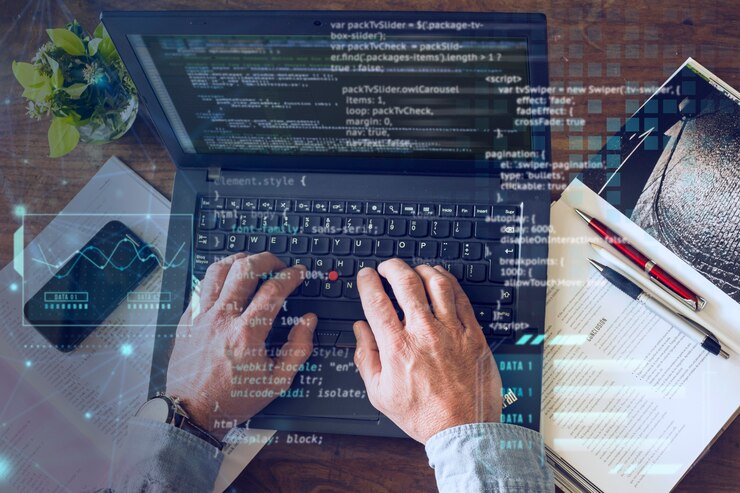
Topic 1: Nested Conditional Statements
Nested conditionals are like decision trees. If the first condition is true, it checks another condition inside it.
Program 1: Meal Decision
# Title: Meal Decision
# Input: Time of day
time_of_day = input("Enter the time of day (morning/afternoon/evening): ")
# Nested conditions to suggest a meal
if time_of_day == "morning":
print("Enjoy your breakfast!")
elif time_of_day == "afternoon":
print("Lunchtime! Grab a bite.")
else:
print("Dinner is served. Bon appétit!")
# Explanation: This program recommends a meal based on the time of day using nested conditionals.
- Input: Time of day (morning/afternoon/evening)
- Explanation: Depending on the time, it suggests a meal. For example, “Lunchtime! Grab a bite.”
Topic 2: Multi-way Decision/ Conditional Statements
In programming, multi-way decision statements are utilized when a situation involves several possible conditions or outcomes. Instead of a binary choice (true or false), multi-way decisions cater to a spectrum of possibilities. These structures empower the program to make nuanced decisions based on various conditions.
Program 2: Traffic Light Simulator
# Title: Traffic Light Simulator
# Input: Traffic light color
traffic_light_color = input("Enter the traffic light color (red/yellow/green): ")
# Multi-way decision to simulate traffic light behavior
if traffic_light_color == "red":
print("Stop! Wait for the green light.")
elif traffic_light_color == "yellow":
print("Caution! Prepare to stop or proceed with care.")
else:
print("Go! The road is clear.")
# Explanation: This program simulates a traffic light's behavior based on the input color.
- Input: Traffic light color (red/yellow/green)
- Explanation: Simulates traffic light behavior based on the input color. For example, “Caution! Prepare to stop or proceed with care.”
Topic 3: else-if Statement vs if-if Conditional Statement
The else-if statement, also known as the “elif” statement in Python, provides a structured way to check multiple conditions sequentially. It operates in a step-by-step manner, examining each condition in order. Once it encounters a true condition, it executes the associated block of code and exits the structure. If none of the conditions is true, the else block (if present) can provide a default action.
Program 3: Exam Result Evaluator
# Title: Exam Result Evaluator
# Input: Exam score
exam_score = int(input("Enter your exam score: "))
# else-if statement to evaluate exam results
if exam_score >= 90:
print("Excellent! You scored an A.")
elif 80 <= exam_score < 90:
print("Good job! You scored a B.")
else:
print("Study harder. You need to improve.")
# Explanation: This program evaluates exam results using an else-if statement.
- Input: Exam score
- Explanation: Evaluates exam results using else-if. For example, “Good job! You scored a B.”
Topic 4: Conditional Expressions
Conditional expressions, a concise programming tool, let you make decisions in just one line. They serve as a shorthand for if-else statements, streamlining code and making it more readable. Instead of several lines of code, you can use a single line to express conditions and assign values based on those conditions. This simplicity enhances code efficiency and is particularly handy for straightforward decision-making in various programming scenarios.
Program 4: Number Comparison
# Title: Number Comparison
# Input: Two numbers
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
# Conditional expression to compare numbers
result = "equal" if num1 == num2 else "not equal"
# Output the result
print(f"The numbers are {result}.")
# Explanation: This program uses a conditional expression to compare two numbers.
- Input: Two numbers
- Explanation: Uses a short expression to compare two numbers. For example, “The numbers are equal.”