In Python programming, knowing how to use Python conditional statements, if statements and other conditions is crucial. This article explains the basics of if statements, if-else statements, nested if statements, and logical conditions. It includes easy-to-understand explanations of Python conditional statements, examples, and tips for better coding. You can run your code on any Python IDE or Google Colab notebook.
If Statement:
- The
if
statement is a fundamental control flow statement in Python. It executes a block of code only when a specified condition is true. - Syntax:
if condition:
# code to be executed if the condition is true
When the specified condition is true, the code inside the indented block executed.
Program 1: Checking Positive Number
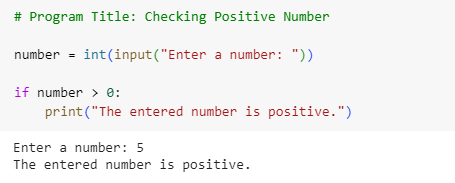
Program 2: Eligibility to Vote
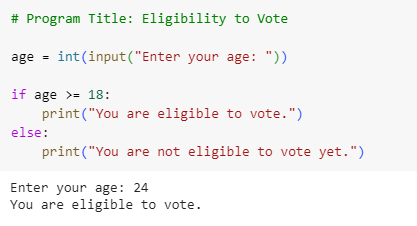
If-Else Statement:
- The
if-else
statement extends theif
statement by providing an alternative block of code to be executed if the condition in theif
statement is false. - Syntax:
if condition:
# code to be executed if the condition is true
else:
# code to be executed if the condition is false
If the condition is true, the code inside the first block will be executed; otherwise, the code inside the else
block will be executed.
Program 1: Freezing Temperature Check
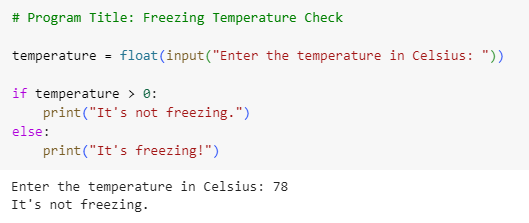
Program 2: Odd or Even Number Check
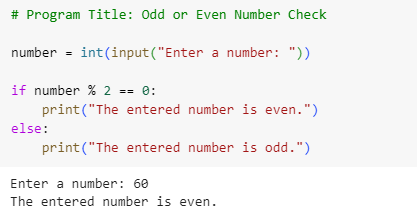
Nested IF Statement:
- You can nest
if
statements within otherif
statements to create more complex decision-making structures. This is useful if you have multiple conditions to check. - Example:
if condition1:
if condition2:
# code to be executed if both conditions are true
Nested if
statements allow you to check multiple conditions, with the inner block being executed only if both the outer and inner conditions are true.
Program 1: Nested Condition Check
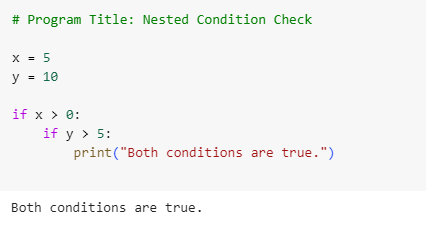
Program 2: Nested Grade Check
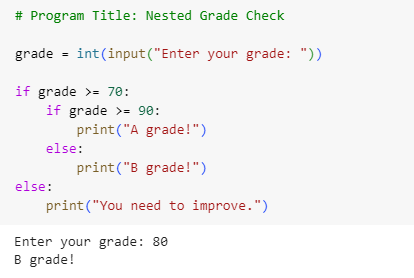
If-Elif-Else Statement:
- When you have multiple conditions to check, you can use if-elif-else statement.
- Syntax:
if condition1:
# code to be executed if condition1 is true
elif condition2:
# code to be executed if condition1 is false and condition2 is true
else:
# code to be executed if both condition1 and condition2 are false
if-elif-else
allows you to check multiple conditions sequentially. The first true condition triggers its corresponding block, and if none are true, the else
block is executed.
Program 1: Grade Evaluation
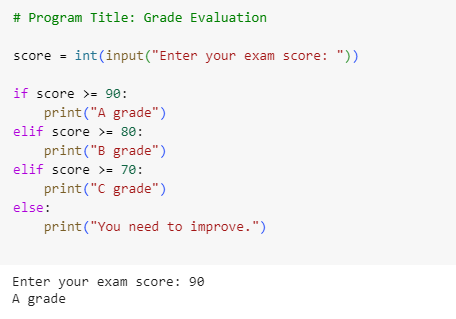
Program 2: Number Sign Check
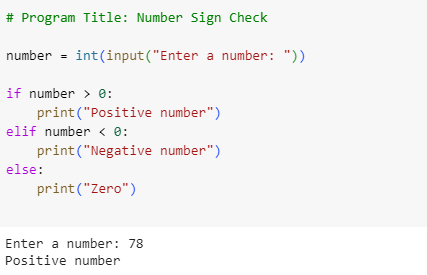
What Happens When the ‘If Condition’ Does Not Meet?
- If the
if
condition is not met and there is noelse
block, the program simply proceeds to the next statement after theif
block.
When ‘Else Condition’ Does Not Work?
- If the
else
condition doesn’t operate, it could stem from a logical error in your code or a situation where the condition in theif
statement consistently evaluates as true.
Logical Conditions in Python:
Equals (==):
- This condition checks if two values are equal.
- Example:
a = 5
b = 5
if a == b:
# Code here executes because a is equal to b
Not Equals (!=):
- This condition checks if two values are not equal.
- Example:
a = 5
b = 10
if a != b:
# Code here executes because a is not equal to b
Less Than (<):
- This condition checks if the value on the left is less than the value on the right.
- Example:
a = 5
b = 10
if a < b:
# Code here executes because a is less than b
Less Than or Equal To (<=):
- This condition checks if the value on the left is less than or equal to the value on the right.
- Example:
a = 5
b = 5
if a <= b:
# Code here executes because a is less than or equal to b
Greater Than (>):
- This condition checks if the value on the left is greater than the value on the right.
- Example:
a = 10
b = 5
if a > b:
# Code here executes because a is greater than b
Greater Than or Equal To (>=):
- This condition checks if the value on the left is greater than or equal to the value on the right.
- Example:
a = 10
b = 10
if a >= b:
# Code here executes because a is greater than or equal to b